Getting Started with DebuggerTypeProxy
The DebuggerDisplay feature in Visual Studio has been introduced before. Another feature similar to this one is named DebuggerTypeProxy, which we will explore it below.
Consider the class below:
public class Data
{
public string Name { get; set; }
public string ValueInHex { get; set; }
}
After executing the program, the values of the created class will be as follows:
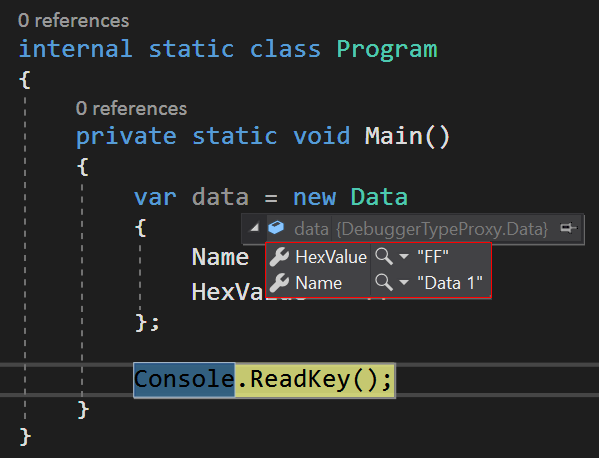
Here, the Hex value is not understandable for us. Imagine a scenario where values need to be stored in the database in Hex format, but during debugging, we want to display the HexValue property in an understandable decimal form.
To do this, we can use the DebuggerTypeProxy feature. First, we create a class that acts as a proxy, displaying the values the way we need them. This class receives the original object in its constructor, and the values we’re interested in are accessible through the properties we define in it:
public class DataDebugView
{
private readonly Data _data;
public DataDebugView(Data data)
{
_data = data;
}
public string DecimalValue
{
get
{
bool isValidHex = int.TryParse(_data.HexValue, NumberStyles.HexNumber, null, out var value);
return isValidHex ? value.ToString() : "INVALID HEX STRING";
}
}
}
Finally, to apply this proxy class, we use the DebuggerTypeProxy attribute on the main class:
[DebuggerTypeProxy(typeof(DataDebugView))]
public class Data
{
public string Name { get; set; }
public string HexValue { get; set; }
}
After making the changes and rerunning the program, the way the class values are displayed during debugging session will change:
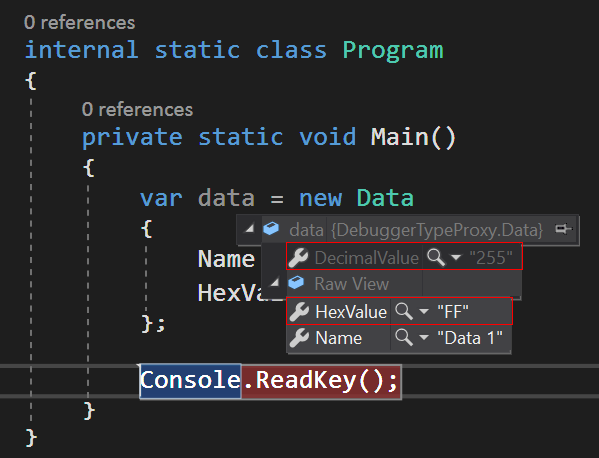